While Loops
Contents
While Loops#
While loops are used to repeat a block of code while a given condition is true. If this condition is false (or becomes false), the code block will not be repeated. I will refer to individual loop repetitions as “iterations” (the first time the code in the loop is run is the first iteration, the next time is the second iteration, etc.).
The syntax for a while
loop is:
while condition:
code block
Note the use of the while
statement and the :
following the condition. All the code indented after the :
is inside the loop, represented by code block
here. The condition
used in the loop must either evaluate to or be a boolean value.
In each loop iteration condition
is evaluated. If condition
is found to be true then the loop will go through another iteration, executing code block
, and checking for another iteration. If condition
is found to be false, then control will leave the loop and it will not undergo another iteration.
Note that if condition
starts as false, then code block
will never be executed.
The while loop can be illustrated with the control flow diagram:
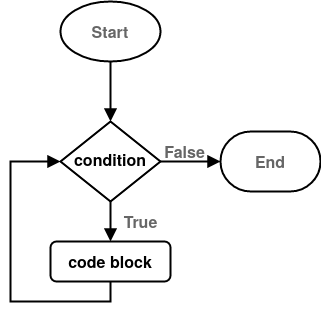
Fig. 13 Control flow diagram of the while loop.#
Worked Example
Let’s consider the following problem where we can make use of a while
loop to solve the recursive series:
Let’s say we want to know when this series drops below 2 (what is the first value of \(n\) for which \(T_n < 2\)). One solution is:
T = 100 #T_0 term
n = 0
while T >= 2:
T = T**(3/4.) #T_{n+1} term
n += 1
print('T_n is less than 2 for n =', n)
T_n is less than 2 for n = 7
Notice how the condition is T >= 2
and not T < 2
. That is because the loop continues while the condition is true and we want the loop to stop when T < 2
is True
(and the converse T >= 2
is False
).
Avoiding Infinite Recursion#
Something to be careful of when using while
loops is a loop that doesn’t stop looping. If condition
never evaluates to False
, or if you never break out of the loop in another way, control will never leave the loop. Sometimes it is useful to use a maximum number of loop iterations to avoid this:
counter = 0
while condition and counter < max_count:
block of code
where max_count
is the chosen maximum number of recursions (normally chosen as a very large number).